As we all know, web development is a very time consuming and difficult task. To simplify the development process, software engineers approach website creation by using Angular or React, the most popular tools for frontend development nowadays. Although it is worth noting that these technologies are very different, it is impossible to say unequivocally which is better – React or Angular.
Main definitions
Angular
Is Angular a framework? Totally yes. It is made by Google for building client applications. When asked why use Angular, first of all, it is aimed at developing SPA solutions. In this regard, Angular came from Angular JS. At the same time, it’s not an update to its predecessor, but a fundamentally new framework.
React
React is an open-source JavaScript library for developing user interfaces. It has been developed and maintained by Facebook, Instagram, and the community of individual developers and corporations. Its goal is to provide high speed, simplicity, and scalability. Moreover, React is often used in tandem with other libraries for developing user interfaces.
Difference between React and Angular
Although both technologies are used for software development in JavaScript, they have significant differences, which we will now consider.
One- and two-way Data Binding
Data binding is a feature that allows you to synchronize data between the application model and the view. In one-way data binding, any change in the state of the application automatically updates the view. In contrast, two-way data binding binds together properties and events under the same object, that is, any modification to the model updates the view and vice versa.
Angular uses two-way data binding, while React uses one-way data binding. In React, properties are passed from parent to child components, which is called one-way data flow, or top to bottom. The component state is encapsulated and not available to other components unless it is passed to the child component as props, i.e. the component state becomes the child component’s props.
But what if you need to propagate data through a component tree? This is done through child events and parent callbacks.
In React, properties are passed from parent to child components, which is called unidirectional data flow, or top to bottom. The component state is encapsulated and not available to other components unless it is passed to the child component as props, i.e. the component state becomes the child component’s props. But what if you need to propagate data through a component tree? This is done through child events and parent callbacks.
The data binding methods available in Angular relate to several features that make this process interesting. Angular has built-in support for interpolation, one-way binding, two-way binding, and event binding. Interpolation is the easiest way to bind a property of your component inside the text between your HTML tags and attribute assignments. Property binding is similar to interpolation in the sense that you can bind the properties of your view elements to the properties of a component. Property binding facilitates the exchange of components and is identical to how props are passed to React. Event binding allows data to flow in the opposite direction, from element to component. But the most important feature is two-way binding using [(ngModel)]. This combines property binding and event binding in one directive and is especially useful for forms and input fields.
Rendering
Server-side processing is a traditional rendering technology. The server returns the entire HTML file upon request, and the browser retains a simple presentation of it to the user. On the other hand, client-side rendering returns an HTML document, stylesheet, and JavaScript file.
JavaScript makes subsequent requests to render the rest of the website using the browser. React or Angular, and all the other modern JavaScript front-end libraries are good examples of client-side rendering. This is visible if you view the source code of your Angular or React application.
But client-side rendering has drawbacks as it is not very SEO friendly and it returns incomplete HTML content when you share your link on social media sites. Angular has a solution called Angular Universal, which will take care of making your app friendly to a search engine and social media. This is a library built by the Angular team and will definitely be useful. Universal uses a pre-rendering technique where the entire site is first rendered from the server, and after a couple of seconds, the user switches to client-side rendering. Since all this happens under the hood, the user doesn’t notice anything.
If you are using React with Redux, the Redux documentation has a good guide on setting up server rendering. You can also configure React to render from the server using the BrowserRouter and StaticRouter components available in the react-router library. But if you are looking for performance and optimization, you can try next.js, which is a library for SSR in React.
DOM
One of the main differences between React vs Angular is that the first one uses the Virtual DOM. And this fact is attributed to the advantages of this framework.
The DOM is a way to represent the content of an HTML document as objects. Also, there is an interface for managing the specified objects.
There is a regular DOM (also called real) and a virtual one. Let’s look at the differences with an example. If you want to change certain information in HTML tags, the algorithms will act as follows: the real DOM will update all tags until it finds the fragment it needs. And this in some cases negatively affects performance and other parameters. The virtual DOM will update only the required HTML chunk.
If you need to work with 20-30 asynchronous data requests on one page, then it would be better to use technology with virtual DOM since otherwise the entire HTML block will be replaced for each page request, and this will affect the performance and user experience of the site.
Typification in Angular or React
A static type check is performed at compile time. The compiler warns you of possible type mismatches and detects some errors that would otherwise go unnoticed. Also, defining a contract by a variable, property, or function parameter can result in a more readable and supported code. Declarations of variables and functions become more expressive by declaring their data types.
Defining the API signature using the interface makes the code less ambiguous and understandable. The interface serves as a quick start-up guide that helps you get started with the code right away and saves time spent reading documentation or implementing the library.
You can use the type keyword in TypeScript to create an alias for a type. You can then create new types that are a union or intersection of these primitive types.
React has limited support for type checking because basic ES6 doesn’t support it. However, you can implement type checking using the prop-types library developed by the React team.
But prop-types are not limited to strings, numbers, and booleans. You can do a lot more as described in the prop-types library documentation. However, if you are serious about static type checking, you should use something like Flow, which is a static type checking library for JavaScript.
Open-source code
React has over 100,000 codes and 300 ready-made solutions for modern problems. React has a time advantage since it was released to the market 3 years before Angular came along. And that means that it faced a lot of real-world problems, passed critical tests, and overall turned into a flexible and user-friendly open-source library loved by many developers.
Angular has 6 times more out-of-the-box solutions to various problems than React, is much more structured, and has fewer developers using it since it was released 3 years later than its opponent.
Comparison of Angular vs React as TypeScript vs JavaScript
When researching React vs Angular, it would be wrong not to mention the languages of their development – TypeScript and JavaScript. If we abstract from the main topic of this article and dwell only on what these languages are, then we can say the following.
JavaScript is a scripting language that follows client-side programming rules. It works in a web browser without the need for a web server or anything else. This allows the code to interact with the browser and can even change or update both HTML and CSS. Although it is possible to use JavaScript with REST API, XML, and others, it is not intended for developing applications, applets, or large complex systems.
JavaScript was originally developed as a client-side programming language, but after a while, developers realized that it could also be used for server-side programming. As JavaScript grew, its code became heavy and complex to the point that it could not even meet the requirements of object-oriented programming.
This limited the further growth of JavaScript as a server-side language. Then TypeScript was born.
Typescript is sort of an updated version of the Javascript language. It can run on Node.js or any web browser that supports ECMAScript 3 or higher. TypeScript is a statically compiled language that provides optional static typing, classes, and interface. It allows you to write simple and clean JavaScript code. Thus, the adoption of TypeScript can help create more easily deployable and more reliable software.
Let’s talk about the difference between these languages.
- JavaScript is an easy-to-learn language, while TypeScript has a tough learning curve and requires prior knowledge of scripting.
- JavaScript is a scripting language and TypeScript is an object-oriented programming language.
- TypeScript supports modules, but JavaScript does not.
- TypeScript supports static typing, which allows you to check the type correctness at compile time, whereas JavaScript does not.
- TypeScript code must be compiled, but JavaScript does not need to it.
- TypeScript supports the optional parameter function, but JavaScript does not.
- JavaScript has a large developer community, but TypeScript does not.
What is better to utilize for your software project: Angular or React
Nowadays, it is necessary to analyze the popularity of any technology to understand whether it’s easy to find a good specialist to hire. For doing this, NPMtrends can be a great help.
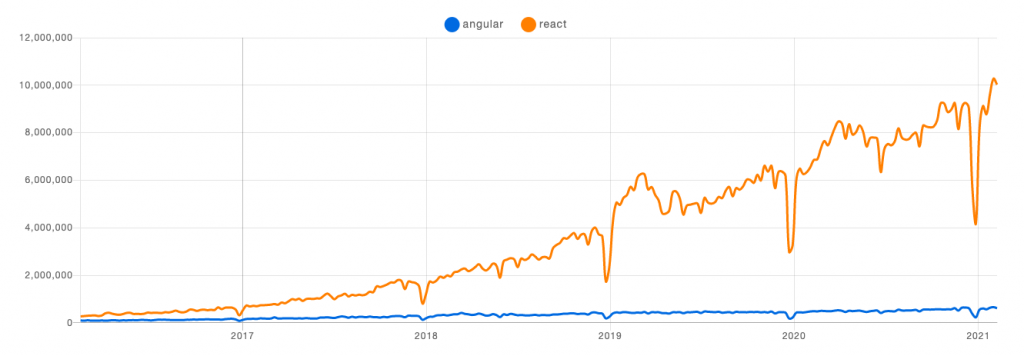
According to statistics over the past two years, it is clear that React prevails over Angular. However, you can also see that with the decline in the number of downloads from React, Angular also begins to sink, which indicates the dependence of one on the other.
According to GitHub statistics, Angular has 69.8K stars, while React has 162K.
If you look at the State of JS research for 2020, you can see the following picture:
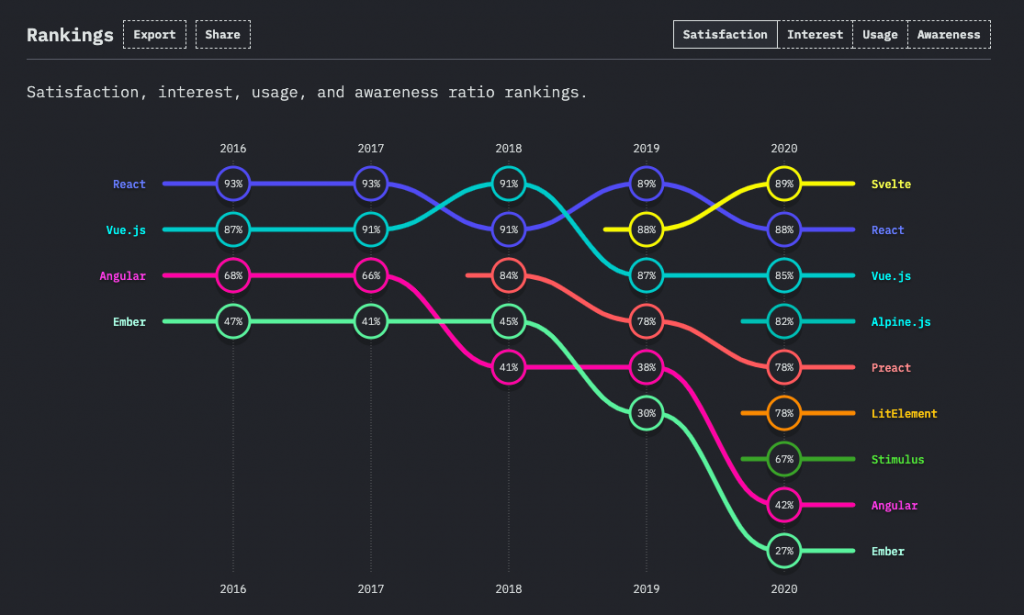
The popularity of React has decreased by only 1% and is 88%, while the popularity of Angular has increased, but still falls short of React and is 42%.
If you look at the developers’ impression of using these technologies, you can see in the following pictures:
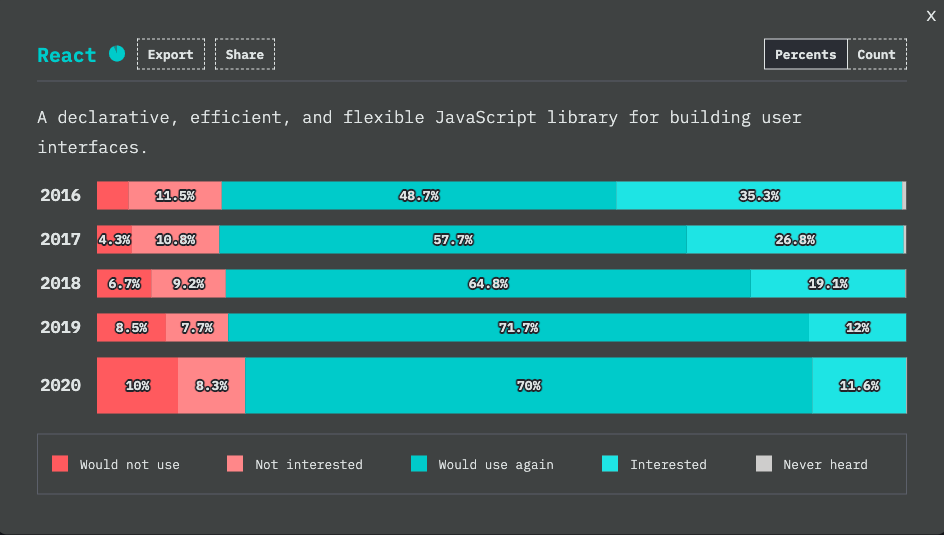
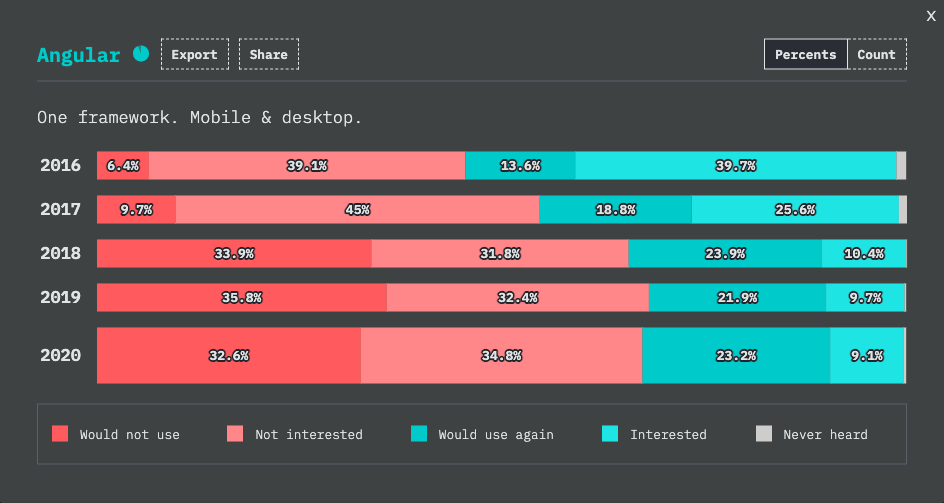
It can be seen that developers who had experience using React have an extremely small percentage of disinterest and unwillingness to work with this technology. Angular has the opposite situation. After mid-2017, the percentage of dissatisfied people using this technology has noticeably increased, but this figure is slowly but surely declining.
Conclusion
Novice developers often wonder which is easier, React or Angular? The answer is clear. Angular is easier to learn. Nevertheless, React provides more possibilities. Based on this, you just need to prioritize and choose what is more suitable for a specific task.
The technology you choose can affect how much time you spend coding and your budget. If you have a team of C#, Java, or C++ developers, then it would be more logical to go to Angular, since TypeScript has a lot in common with these languages and will be easier with it.
As an experiment, you can create an application in both Angular and React, then evaluate the language, usability, and performance, and make a decision. Both technologies have their own set of advantages and similarities, and it comes down to what functionality the app will offer.
That is why, if your business has an urgent need to develop certain software, the best solution would be to trust an experienced team that works with these technologies and can tell you exactly which one is the best for your needs. Such a development team can be found at Bamboo Agile. If you already know exactly what you need or you just have an idea, then feel free to fill out the form on our website https://bambooagile.eu/contact-us or write directly to us at contact@bambooagile.eu to discuss the details of your future project.