Introduction
Spring Framework has become noticeably more complex over time. The gradual build-up of extra functionality is to thank for that. Now, starting a new project requires a long setup process.
But not all is lost. Spring Boot was designed specifically to combat this problem – and it does so with flying colours. Today, we will talk all about this tool and how it compares to the raw Spring Framework.
What is the Spring Framework?
Spring is a popular open-source framework for developing enterprise applications. It was created to simplify app development on the popular Java EE technology stack from Oracle, which was very complex and difficult to use at the time.
The framework has a robust programming and configuration model. Unlike many other frameworks, it focuses on several areas of application functionality and provides a wide range of additional functions for them.
One of the main advantages of the Spring Framework is its use of the Dependency Injection pattern. DI makes it much easier to implement the functionality that applications need, and allows to develop loosely coupled, more general classes.
Advantages of Spring Framework
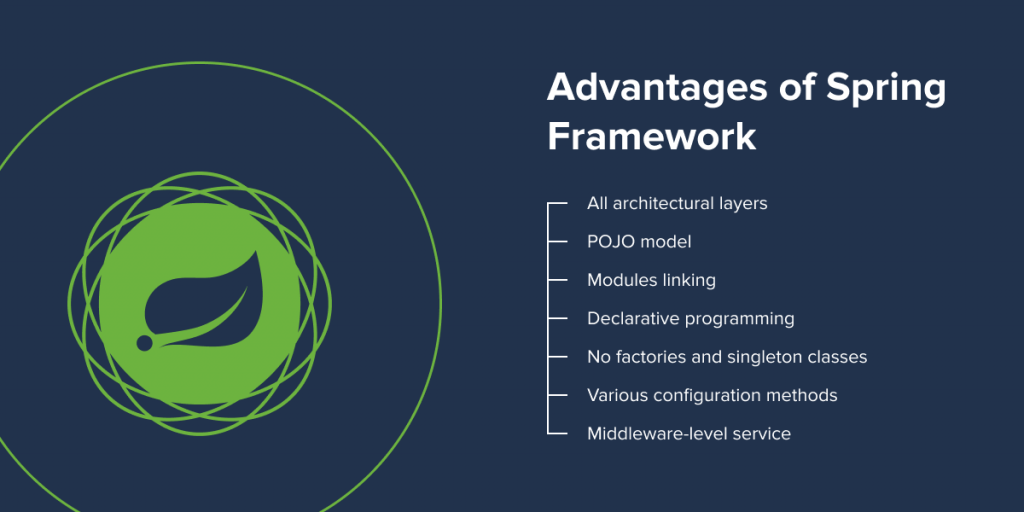
Let’s look at some advantages of Spring Framework:
- Can be employed on all architectural layers of web applications;
- Uses the very lightweight POJO model when writing classes;
- Allows you to freely link modules and easily test them;
- Supports declarative programming;
- Eliminates the need to independently create factory and singleton classes;
- Supports various configuration methods;
- Provides middleware-level service.
In short, the framework offers a lot of benefits. But it does have the downside of a lengthy configuration process. This has contributed to the creation of Java Spring Boot.
What is Spring Boot?
Spring Boot is an open-source framework designed to help with Spring development. It automates configuration and speeds up the process of creating and deploying Spring applications.
While its parent framework focuses on providing flexibility, Spring Boot seeks to reduce code length and simplify web application development. By leveraging annotation and boilerplate configuration, the tool reduces the time it takes to develop apps. This helps you make standalone applications with less or almost no configuration overhead.
Is Spring Boot Popular?
Yes. According to a report by JRebel, 62% of respondents used Spring Boot as their main framework in 2021. It also states that almost 50% of surveyed developers defaulted to working with microservices, the architecture Spring Boot is perfect for.
Read this article to learn all about microservices architecture and how it compares to monolith.
It bears mentioning that its popularity has slightly diminished over the years. This is due to the gradual rise of alternatives, such as Quarkus, Node.js, Django, Micronaut, etc. Even still, the technology remains very relevant in 2023. And will likely stay that way for years to come.
When to Use Spring Boot?
Spring Boot is optimal for building scalable microservices. But it can also be used for developing a broad variety of applications:
- Microservices;
- Utilities;
- MVC Web apps;
- Reactive Web apps;
- Serverless applications;
- Asynchronous applications;
- Mobile backends;
- And more.
In general, the framework makes it easy to create different types of apps with minimal effort. Let’s have a closer look at its unique feature set.
Spring Boot Features
Dependency Management
Managing dependencies is easy with Spring Boot. The framework implicitly packages the required third-party dependencies for each type of application. It then provides them through so-called starter packages (spring-boot-starter-web, spring-boot-starter-data-jpa, etc.).
Starter packages are collections of handy dependency descriptors that you can include in your application. They allow you to get a universal solution for all Spring-related technologies. So you don’t even need to search for code examples or compatible drivers and libraries online.
For instance:
- If you want to start using Spring Data JPA to access your database, just include the spring-boot-starter-data-jpa dependency in your project. That’s it, you’re done.
- If you want to create a web application, just add the spring-boot-starter-web dependency. It will pull all the libraries you need to develop MVC applications into your project, such as spring-webmvc, jackson-json, validation-api, and Tomcat.
Basically, it collects all common dependencies and defines them in one place. As a result, the pom.xml file will contain much fewer lines when used in Spring Boot than in regular Spring.
Automatic Сonfiguration
Another notable feature of the technology is automatic configuration. After choosing a suitable starter package, the software will try to automatically configure your application based on the jar dependencies you added.
For example:
- If you add Spring-boot-starter-web, the system will automatically configure registered beans such as DispatcherServlet, ResourceHandlers, and MessageSource.
- If you are using spring-boot-starter-jdbc, it automatically registers the DataSource, EntityManagerFactory, and TransactionManager beans. It also auto-reads the database connection information from the application.properties file.
But let’s say you’re not going to use a database. Let’s say you don’t provide any details about connecting manually. In that case, the framework will automatically configure the database in the memory without any additional configuration on your part (if you have H2 or HSQL libraries).
And you’re not forced to roll with it. Auto configuration can be completely overridden at any time with user preferences.
Built-in Web Server
Every Spring Boot application includes an embedded web server. This means you no longer have to worry about setting up a servlet container. Instead, the app can run itself as an executable .jar file using the built-in server. And if you need to use a separate HTTP server, simply exclude the default dependencies. The framework provides various starter packages for different HTTP servers.
Microservices Made Easy
You can package an app into a separate .jar file with a full Tomcat container embedded. This feature was borrowed from the Play framework’s application deployment model (though, Spring Boot can also create traditional .war files). Basically, you can quickly package an entire service (e.g. user authentication) into a standalone and fully deployable artefact with an API. This makes installing and deploying software much easier.
Streamlined Testing
You can speed up the QA process by using intuitive default settings for unit and integration tests. Microservices testing is particularly easy with the help of the JUnit testing framework and the @Test annotation. To test only certain parts of the functionality, developers can use the @SpringBootTest annotation followed by the list of components that need to be tested.
Want to make your testing more efficient? Check out our complete tutorial on backend testing.
New Features in Spring Boot 3.0
Spring Boot 3.0 is the newest version of the software, released in November 2022. It introduced a number of new features that deserve special mention.
Java 17 Baseline and Java 19 Support
Spring Boot 3.0 requires at least the 17th version of JDK to work, and it is not compatible with JDK 8 or 11. So if you’re thinking of upgrading to 3.0, make sure to update your version of JDK as well. The software is also compatible with JDK 19.
GraalVM Native Image
3.0 introduced Native Image support. This allows you to convert your application directly into a GraalVM native image. In other words, the feature lets devs create native executables through a static Ahead-of-Time (AOT) compilation using the GraalVM Native Image compiler. This significantly improves application start time and memory consumption.
Java Champion Josh Long says, “…Java hasn’t been the most memory-efficient language. This has foreclosed on some opportunities like IoT and serverless. AOT compilation with GraalVM Native Image puts it in the running while retaining Java’s vaunted scalability and productivity.”
Easier @ConstructorBinding
You don’t need to use the @ConstructorBinding annotation anymore if a @ConfigurationProperties class has a single parameterized constructor. Though, if there are multiple constructors in the class, you still need to employ @ConstructorBinding to specify which one Spring Boot should use.
Log4j2 Extensions
3.0 came with several has introduced new extensions for Log4j2, which include:
- Profile-specific Configuration
- Log4j2 System Properties
- Environment Properties Lookup
All of these radically improve the flexibility of Log4j2, giving developers more room for precise configurations.
Spring Framework 6.0 and Jakarta EE 9
Spring Boot 3.0 is built on Spring Framework 6.0 and Jakarta EE 9. The latter is very significant, since you will have to use the jakarta.* packages instead of javax.* ones. So if your app utilises Jakarta EE types such as annotations, validations, or JPA, you will need to replace all of them with their jakarta.* package equivalents.
Spring Observibility
Spring Observability is part of the Spring Framework 6.0 feature set. The functionality is based on Micrometer and Micrometer Tracing. It allows you to log various software metrics and provides tracing support with OpenZipkin and OpenTelemetry.
Deprecations
However, version 3.0 also came with some deprecations. Namely:
- No more support for cookie comment properties.
- Deprecated the JsonMixinModule scanning-based constructor.
- Deprecated the no-args constructors on HealthContributor @Configuration base classes.
You can find more in the official Spring Boot 3.0 release notes.
Advantages of Spring Boot
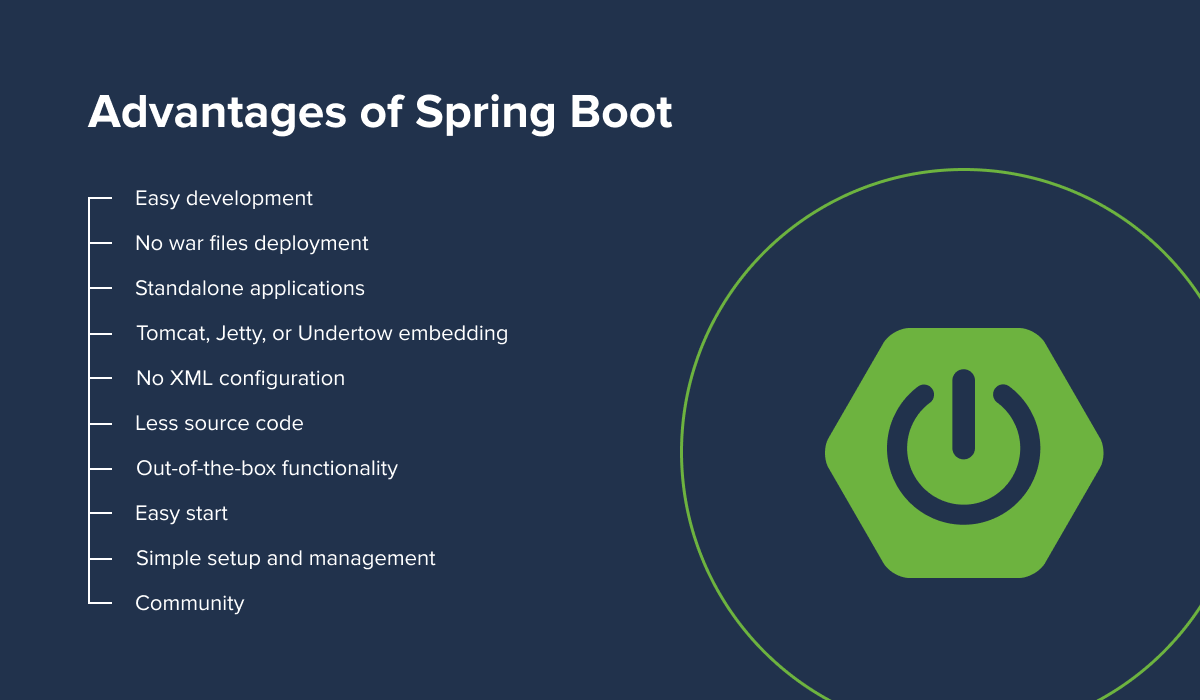
According to Stackshare, the top 3 pros of Spring Boot have been voted to be:
- powerful and handy;
- easy setup;
- operates on Java (which is highly popular).
Generally speaking, Spring Boot helps developers keep things simple when working with Spring. Let’s have a more in-depth look at how it achieves that:
- Easy development. All the framework’s features aim to make development faster and easier;
- No .war files deployment. There’s no need for the deployment of .war files, since you can package apps as .jar executables;
- Standalone applications. You can package services as standalone applications;
- Tomcat, Jetty, or Undertow embedding. You can directly embed Tomcat, Jetty, or Undertow into an application;
- No XML configuration. Automatic configuration removes the need for XML configuration;
- Less source code. The technology reduces the amount of code necessary to write a Spring app;
- Out-of-the-box functionality. The framework comes with a lot of useful features for testing, Maven and Gradle support, an embedded web server for every app, etc.;
- Easy start. It’s is very beginner friendly and sets up a lot of the things you need automatically;
- Simple setup and management. Automated configuration, as well as simple database and dependency management make setup extremely fast;
- Community. Large community and many training programs to facilitate the familiarisation period.
The development team is also very responsive. Josh Long says, “We pay special attention to StackOverflow tags and do our best to respond to them, but if a bug is discovered there, it ultimately lands in GitHub. GitHub is a great way to impact the projects. We try to make it easy, like having labels for newcomers who want to contribute to start somewhere where we could mentor them.”
All in all, the framework does an incredible job of simplifying Spring development. It streamlines dependencies and allows users to run applications directly from the command line. It also doesn’t require an external application container and greatly reduces the amount of code necessary for configuration.
Disadvantages of Spring Boot
In spite of the many advantages of Spring Boot, it still has a couple of drawbacks that you should keep in mind:
- Lack of control. The system creates a lot of unused dependencies, resulting in a large deployment file;
- Hard to convert to. The process of converting a legacy or an existing Spring project to a Spring Boot application can be complex and time-consuming;
- Not suitable for large-scale projects. Many developers claim the tool is not suitable for building big monolithic applications. Though some argue that it can handle large projects just fine, it’s far from a common consensus;
- Can be inflexible. Spring Boot uses an opinionated approach. This means it gravitates towards a single solution to any given issue. It reduces decision making in the coding process, but also makes it less flexible than that of the parent framework.
Spring Framework vs. Spring Boot
Now that we know all about the Spring Framework and Spring Boot, let’s compare the two.
Spring Framework | Spring Boot | |
Technology | Open-source Java framework | Spring Framework extension |
Purpose | Provides a flexible environment with libraries and tools for building web apps | Provides similar features, but uses less code and lets apps run without annotations or XML configuration |
Key feature | Dependency injection | Autoconfiguration |
Embedded servers | No | Yes (Tomcat, Jetty) |
Configuration | Manual | Automatic |
XML knowledge | Required | Not required |
CLI tools | No | Yes |
Approach | Unopinionated | Opinionated |
Use cases | Enterprise solutions, apps with unique configurations, apps that require lots of flexibility, etc. | Microservices, REST APIs, small applications, serverless apps, etc. |
All in all, the choice depends on your preferred coding style and the type of application you wish to create.
FAQ
What is Spring Boot used for?
Spring Boot is used to simplify software development with the Spring framework.
Is Spring Boot a testing tool?
Spring Boot isn’t specifically meant to be a testing tool, but it can be used as such. It’s especially great for microservices testing, where developers can employ the JUnit testing framework and the @Test annotation.
Is Spring Boot full stack?
Yes, it’s widely used both for frontend and backend development.
Does Spring Boot use SQL?
Yes, Spring Boot supports a large range of SQL databases. It can also automatically configure embedded H2, HSQL, and Derby databases.
Conclusion
Spring Boot is an integral part of the Java ecosystem. It offers an efficient and scalable toolbox for building Spring applications with a microservices architecture. Carefully review how its pros and cons align with your business goals to know if this framework is right for you.
Alternatively, consult a reliable software vendor on which technology would be best for your project. Bamboo Agile has years of experience with Java and can become your trusted partner on this journey.
Sign up for a free consultation with our specialists. We’ll be happy to answer your inquiries!